The node editor allows you to build GLSL shaders visually. Instead of writing code, you can create and connect nodes in a graphical framework. The node editor instantly displays your changes giving instant feedback. Is simple enough for users new to creating shaders.
Procedural shaders using Node Editor
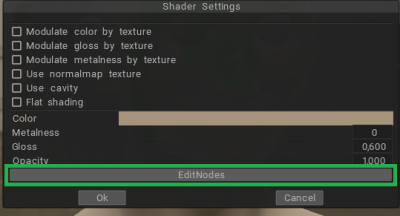
Node editor description
A Node defines an input, output, or operation on the Shader editor, depending on its available Ports. A Node may have any number of input and/or output Ports. You create a graph by connecting these Ports with Edges. A Node might also have any number of Controls; there are controls on the Node that do not have Ports.
You can collapse a Node by clicking the Collapse button in the top-right corner of the Node. This will hide all unconnected Ports.
The components of a Node are the port & edge
Port
A Port defines an input or output on a Node. Connecting Edges to a Port allows data to flow through the Shader Graph node network.
Each Port has a Data Type that defines what Edges can be connected to. Each Data Type has an associated color for identifying its type. Only one Edge can be connected to any input Port, but multiple Edges can be connected to an output Port.
You can open a contextual Create Node Menu by dragging an Edge from a Port with the left mouse button and releasing it in an empty workspace area.
Default Inputs: Each Input Port, a Port on the left side of a Node implying that it is for inputting data into the Node, has a Default Input. This is a small field connected to the Port when no Edge is connected. This field will display input for the port’s Data Type unless the Port has a Port Binding.
If a Port does have a Port Binding, the default input field may display a special field, such as a dropdown for selecting UV channels, or just a label to help you understand the intended input, such as coordinate space labels for geometry data.
Edge
An Edge defines a connection between two Ports. Edges define how data flows through the Shader editor node network. They can only be connected from an input Port to an output Port.
Each Edge has a Data Type that defines what Ports it can be connected to. Each Data Type has an associated color for identifying its type.
You can create a new Edge by clicking and dragging from a Port with the left mouse button. Edges can be deleted with Delete (Windows), Command + Backspace (OSX), or from the context menu by right-clicking on the Node.
You can open a contextual Create Node Menu by dragging an Edge from a Port with the left mouse button and releasing it in an empty workspace area.
There are many available Nodes in Shader Graph. For a full list of all available Nodes see the Node Library.
Tutorial
Make procedural shaders for Sculpt objects using Node graph editor by Alexn007.
Bake shader node
Once you get your procedural shader, this is the command used to bake procedural to geometry:
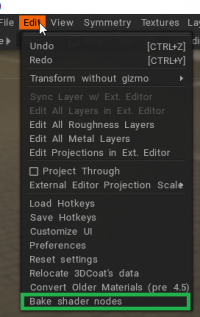
Bake shader nodes: Bake procedural mesh deformers and materials from the Node editor to the real color/displacement. It may be helpful for the export.
Clear
Clear the shader editor panel leaving it empty.
Blend
Sum: Returns the sum of the two input values.
Subtraction: Returns the result of input A minus input B.
Average: This node will average grayscale inputs. Each input can be individually weighted.
Mix: Mixture of input A and input B.
Divide: Returns the result of input A divided by input B.
Abs: Returns the absolute value of input In.
Clamp: Returns the input In clamped between the minimum and maximum values defined by inputs Min and Max, respectively.
Max: Returns the largest of the two input values, A and B.
Min: Returns the smallest of the two input values, A and B.
Round: Returns the value of input In rounded to the nearest integer or whole number.
Saturate: Returns the value of input In clamped between 0 and 1.
Smoothstep: Returns the result of a smooth Hermite interpolation between 0 and 1 if input In is between inputs Edge1 and Edge2.
Step: Returns 1 if the value of input In is greater than or equal to the value of input Edge; otherwise, returns 0.
Trunc: Returns the integer, or whole number, component of the value of input In.
Multiplication: Returns the result of input A multiplied by input B.
Geometry
ACos: Returns the arccosine of each component the input In as a vector of equal length.
ASin: Returns the arcsine of each component the input In as a vector of equal length.
ATan: Returns the arctangent of the value of input In. Each component should be within the range of -Pi/2 to Pi/2.
Cos: Returns the cosine of the value of input In.
Cosh: Returns the hyperbolic cosine of input In.
Cross: Returns the cross product of the inputs A and B values.
Distance: Returns the Euclidean distance between the inputs A and B values.
Dot: Returns the dot product, or scalar product, of the values of the inputs A and B.
Length: Returns the length of input In.
Normalize: Returns the normalized vector of input In.
Reflect: Returns a reflection vector using input In and a surface normal Normal.
Refract: Returns a refraction vector using input In and a surface normal Normal.
Sin: Returns the sine of the value of input In.
Sinh: Returns the hyperbolic sine of input In.
Tan: Returns the tangent of the value of input In.
Tanh: Returns the hyperbolic tangent of input In.
Math
Ceil: The ceiling returns the smallest integer value or a whole number greater than or equal to the value of input In.
Exp: Return the exponential value of input In.
Exp2: Return 2 raised to the power of the parameter.
Floor: Return the largest integer value or whole number that is less than or equal to the value of input In.
Mod: Modulo returns the remainder of input A divided by input B.
Fract: Fraction returns the fractional (or decimal) part of input In, which is greater than or equal to 0 and less than 1.
Log: Return the logarithm of input In.
Log2: Return the base 2 logarithm of the parameter.
Mul: Multiply returns the result of input A multiplied by input B.
Pow: Return the result of input A to the power of input B.
Inverse sqrt: Return the result of 1 divided by the square root of input In.
Sign: Return -1 if the value of input In is less than zero, 0 if equal to zero, and 1 , if greater than zero.
Sqrt: Return the square root of input In.
Textures
ndFilePath: Open file path selection to assign a texture.
ndSampler2D: A sampler2D is used to do a lookup in a standard texture image; a samplerCube is used to do a lookup in a cube map texture.
The value of a sampler variable is a reference to a texture unit. The value tells which texture unit is invoked when the sampler variable is used for texture lookup.
UV Texture: Assign clamp and smooth values to UV texture.
TriPlanarTexture: A method of generating UVs and sampling a texture by projecting in world space.
Effects
Curve: Assign values by curve deform graph.
Invert: Inverts the colors of input In on a per-channel basis. This Node assumes all input values are in the range 0 – 1.
Convert
To Vector: Convert RBGA values to the greyscale channel.
To Channels: Convert Greyscale values to RGBA channel.
Degrees: Returns the value of input In converted from radians to degrees. One radian equals approximately 57.2958 degrees, and a full rotation of 2 Pi radians equals 360 degrees.
Radians: Returns the value of input In converted from degrees to radians.
One degree is equivalent to approximately 0.0174533 radians, and a full rotation of 360 degrees is equal to 2 Pi radians.
Patterns2D
BrickPattern: The Brick pattern adds a procedural texture producing bricks.
SwirlyPattern: The Swirly pattern adds a procedural texture, producing a swirl.\ VonoiPattern: The Voronoi Texture node evaluates a Worley Noise at the input texture coordinates.
CMYKHalftonePattern: Halftoning is also commonly used for printing color pictures. The general idea is the same, by varying the density of the four secondary printing colors, cyan, magenta, yellow, and black (abbreviation CMYK), any particular shade can be reproduced.
Mountains: Is used to add a procedural texture producing Fractal Brownian motion to create a fractal-looking pattern.
Ocean: This is a flexible shader for creating seas, oceans, rivers, and other water surfaces.
Patterns3D
HardNoise3D: Generates a gradient, or Perlin, noise based on input UV.
Celular3D: Generates a Celular noise based on input UV.
InverseSphericalFibonacci: Generates yield nearly uniform point distributions on the unit sphere.
Voronoi3D: Generates a Voronoi, or Worley, noise based on input UV.
SoftNoise3D: Generates a simple, or Value, noise based on input UV.
AVObjects
AVPlane: A class for generating plane geometries.
AVSphere: Sphere is a geometry class for generated spheres with a given ‘Ray position’ and ‘Radius’.
AVBox: Box is a geometry class for a rectangular cuboid with a given ‘position’ and ‘size’.
AVEllipsoid: is a geometry class for an ellipse with a given ‘position’ and ‘size’.
AVTorus: A class for generating torus geometries.
AVCappedTorus: A class for generating modified torus geometries.
AVHexPrism: The hexagonal prism is a prism with a hexagonal base.
AVCapsule: Capsule is a geometry class for a capsule with a given radius and height.
AVRoundCone: A class for generating cone geometries with a rounded base.
AVEquilateralTriangle: A class for generating Equilateral Triangle geometries.
AVTriPrism: A triangular prism is a three-sided prism; it is a polyhedron made of a triangular base.
AVCylinder: A class for generating cylinder geometries.
AVCylinderArbitrary: A class for generating tube geometries.
AVCone: A class for generating cone geometries.
AVConeDot: A class for generating cone geometries.
AVConeD: A class for generating cone geometries.
AVSolidAngle: A solid angle is a measure of the amount of the field of view from some particular point that a given object covers. It measures how large the object appears to an observer looking from that point.
AVOctahedron: A class for generating an octahedron geometry.
AVPryramid: A pyramid is a geometry whose outer surfaces are triangular and converge to a single step at the top.
GlobalIO
IOTime: Provides access to various Time parameters in the shader.
IOMouse: This shader changes color wherever you click on the board, adjusting the color based on mouse position.
IOLightDir: Modify the shader to have specular lighting in it.
IOIteration:
IOCameraPosition: Provides access to various parameters of the current Camera.
GeometryIO
ioUV: Provides access to the mesh vertex or fragment’s UV coordinates.
ioFragCoord: This is an input variable that contains the window relative coordinate for any location within the pixel or one of the fragment samples.
ioPosition: Provides access to the mesh vertex or fragment’s Position.
ioNormal: Provides access to the mesh vertex or fragment’s Normal Vector.
MaterialIO
IODisplacement: Unlike bump mapping, which is a shading effect and does not create actual geometry, displacement mapping correctly generates new geometry from a base mesh and specifies displacement map by displacing the mesh vertices along their normals according to the displacement map.
IOCavity: Cavity maps are a black and white mask that will give you access to crevices and high-frequency details on your model.
IOOcclusion: An invisible material that hides objects rendered behind it.
IOAlbedoColor: Albedo can be considered a basic “reflectivity color” for a material.
IOReflectionColor: This is the reflection of light or other waves or particles from a surface such that a ray incident on the surface is scattered at many angles rather than at just one angle, as in the case of specular reflection.
IOEmissive: This parameter specifies the base amount of light a material emits (in units of Lumens).
IOMetalness: This is a black-and-white texture that acts as a mask that defines areas on a texture set or material that behave like a metal (white) and do not (black).
IOGloss: The glossy transparent material is a generalization of the specular material to allow non-perfect (i.e., rough) reflection and refraction.
IOOpacity: Float in the range of 0.0 – 1.0, indicating how transparent the material is. A value of 0.0 indicates fully transparent, and 1.0 is fully opaque.
Variables
GetLight: Provides access to the Scene’s Ambient color values.
FloatVariable: Defines a Float value in the shader. If Port X is not connected with an Edge, this Node defines a constant Float.
IntVariable: Integer defines a constant Float value in the shader using an Integer field.
ColorVariable: Defines a constant Vector 4 value in the shader using a Color field.
Transform: Transformation of vertices of primitives (e.g., triangles) from the original coordinates (e.g., those specified in a 3D modeling tool) to screen coordinates.